Create a app that show the map,and can zoom in zoom out(no need java code)
get the SHA 1 Key before access the google map API
SHA1: B2:95:30:42:A3:1C:60:84:BD:67:A9:58:52:FB:68:86:7E:B4:24:7D
SHA1: B2:95:30:42:A3:1C:60:84:BD:67:A9:58:52:FB:68:86:7E:B4:24:7D
use to identify your application
The step we will do later
Go to the google develop conole set first
Create a Billing Account
Create a Project in Developer Console
than enable the Google android api
click enable
set up the 憑證
follow the step to create ,and enter the SHA1
we get the 憑證 finally!!!
AIzaSyBS4eEh9nl3Q051fxwcKajNkVJ5beLr7qg
AIzaSyBS4eEh9nl3Q051fxwcKajNkVJ5beLr7qg
Create and Configure the App
Final
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="pom2.poly.com.myfirstmapp">
<uses-feature
android:glEsVersion="0x00020000"
android:required="true" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="AIzaSyBS4eEh9nl3Q051fxwcKajNkVJ5beLr7qg" />
<meta-data
android:name="com.google.android.gms.version"
android:value="@integer/google_play_services_version" />
</application>
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
</manifest>
|
compile 'com.google.android.gms:play-services:8.4.0'
|
try to Adding Map Fragment to the UI
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<fragment xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/map"
android:name="com.google.android.gms.maps.MapFragment"
android:layout_width="match_parent"
android:layout_height="match_parent" />
|
It can show the amp noe:))))
Configuring the Map with XML
<fragment xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:map="http://schemas.android.com/apk/res-auto"
android:id="@+id/map"
android:name="com.google.android.gms.maps.MapFragment"
android:layout_width="match_parent"
android:layout_height="match_parent"
map:cameraBearing="112.5"
map:cameraTargetLat="40.7484"
map:cameraTargetLng="-73.9857"
map:cameraTilt="65"
map:cameraZoom="17" />
|
cameraBearing is the look direction,0 is north,180 is south
cameraTilt is the see angle
0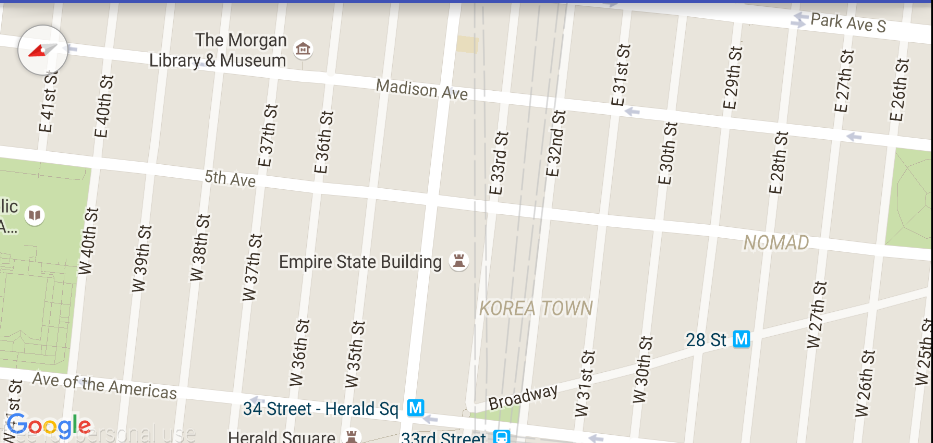
65
the code is here:https://github.com/udacity/google-play-services/tree/master/MapsLessons_Final/Maps3_1
Create a app that can show a map,have different mode(Map,satelite,Hybird),need java code
set the app
like before
set the UI
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:map="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/btMap"
android:layout_width="0"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Map" />
<Button
android:id="@+id/btSatelite"
android:layout_width="0"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Satelite" />
<Button
android:id="@+id/btHybird"
android:layout_width="0"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Hybird" />
</LinearLayout>
<fragment
android:id="@+id/map"
android:name="com.google.android.gms.maps.MapFragment"
android:layout_width="match_parent"
android:layout_height="match_parent"
map:cameraBearing="112.5"
map:cameraTargetLat="22.4081927"
map:cameraTargetLng="113.9799578"
map:cameraTilt="65"
map:cameraZoom="17" />
</LinearLayout>
|
get the GoogleMap object
Main activity implement implements OnMapReadyCallback,and override the onMapReady method,when the map is readt,the onMapReady call,we get the GoogleMap object here
public class MainActivity extends AppCompatActivity implements OnMapReadyCallback {
private boolean mapReady;
private GoogleMap m_map;
@TargetApi(Build.VERSION_CODES.HONEYCOMB)
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
MapFragment mapFragment = (MapFragment)(getFragmentManager().findFragmentById(R.id.map));
mapFragment.getMapAsync(this);
}
@Override
public void onMapReady(GoogleMap googleMap) {
Log.d("map", "onMapReady");
mapReady = true;
m_map = googleMap;//move the camera
LatLng tm = new LatLng(113.9799578, 22.4081927);
CameraPosition target = CameraPosition.builder().target(tm).zoom(40).build();
m_map.moveCamera(CameraUpdateFactory.newCameraPosition(target));
}
}
|
make the buuton code,can show different type of map
public void onClick(View v) {
switch (v.getId()) {
case R.id.btHybird:
m_map.setMapType(GoogleMap.MAP_TYPE_HYBRID);
break;
case R.id.btMap:
m_map.setMapType(GoogleMap.MAP_TYPE_NORMAL);
break;
case R.id.btSatelite:
m_map.setMapType(GoogleMap.MAP_TYPE_SATELLITE);
break;
}
}
|
m_map.setMapType use to set what type of map to show
Depper with google map
Camera Position
zoom:0(whole world) to 21
bearing,the direction of the camera, E90 S180 W270 N0
Tilt is the angle of the camera
the code to move a camera
Different between moveCamera and animateCamer
animateCamer will have animation when move
Create a map that have marker on the map
create the Marker
LatLng home = new LatLng(22.4042335, 113.9808388);
homeMarker=new MarkerOptions().position(home).title("My Home");
|
add the MarkerOption
m_map.addMarker(homeMarker);
|
Customer the icon
BitmapDescriptor icon = BitmapDescriptorFactory.fromResource(R.mipmap.ic_launcher);
homeMarker = new MarkerOptions().position(home).title("My Home").icon(icon);
|
Draw the Polyline on the map
A polyline is a list of points, where line segments are drawn between consecutive points.
GoogleMap map;
// ... get a map.
// Add a thin red line from London to New York.
Polyline line = map.addPolyline(new PolylineOptions()
.add(new LatLng(51.5, -0.1), new LatLng(40.7, -74.0))
.width(5)
.color(Color.RED));
|
Draw the Circle on the map
GoogleMap map;
// ... get a map.
// Add a circle in Sydney
Circle circle = map.addCircle(new CircleOptions()
.center(new LatLng(-33.87365, 151.20689))
.radius(10000)
.strokeColor(Color.RED)
.fillColor(Color.BLUE));
|